Web SDK v2 v3Improve this page
The Fidel SDKs provide a secure way for you to add card-linking capabilities to your applications. The alternative involves using the Fidel Cards API, but you'll need to be PCI Compliant before you can use it. Using the Fidel SDKs, card details are sent directly to the Fidel API through a secure connection without exposing your servers to sensitive information. They take care of all PCI Compliance requirements, so you don't have to.
The Fidel Web SDK v2 is a JavaScript-based SDK that injects a secure HTML iframe into your web page. Your users will need to have JavaScript enabled in their browsers.
The SDK provides a pre-built UI that allows you to easily collect credit card details, tokenise and link credit/debit cards with rewards services from your website, e-commerce platform or mobile web apps. However, for mobile apps, we recommend using the iOS, Android or React Native SDKs.
You can customize the UI of the SDK to match your use case. All modern desktop and mobile browsers are supported, including Chrome, Firefox, Safari, Microsoft IE and Edge.
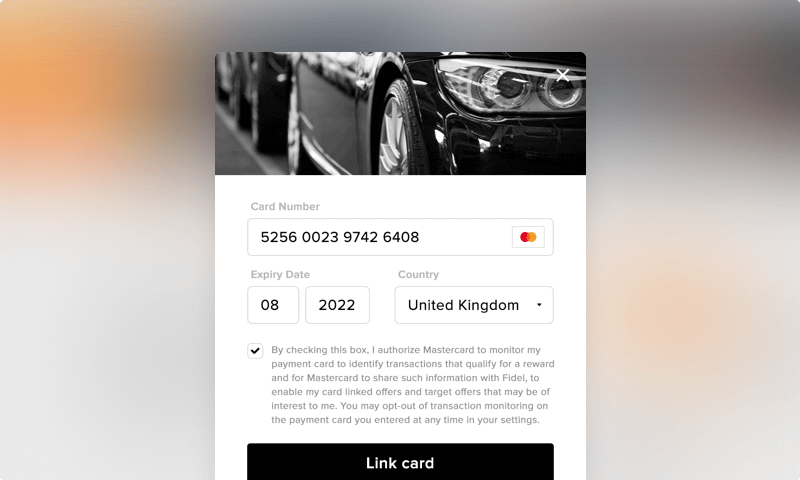
After successfully tokenising and linking a card on the Visa, Mastercard or American Express networks, the Fidel API returns the created Card object. The Card object has a unique id
that you can use to link each unique card and transaction to your user's account. The id
of each linked card is present on the Transaction object as well, as cardId
. You can create card-linked web and mobile applications with online and offline transaction data visibility in a matter of minutes.
Integrating the Web SDK
Integrating the Fidel Web SDK into your web application starts with loading the JavaScript file we provide, https://resources.fidel.uk/sdk/js/v2/fidel.js
, in a <script>
tag. It uses the data-
attributes to allow you to configure it. You can see an example implementation for integrating the Fidel WebSDK in our React sample application on GitHub.
Fidel Web SDK <script>
Configuration
You can configure the Fidel Web SDK by defining attribute parameters on the <script>
tag in your HTML. All of the parameters follow a naming convention and begin with data-
. data-program-id
, data-key
and data-company-name
are the required parameters.
The Fidel Web SDK can be customized to better fit your website. You can provide a button title, title, subtitle and logo by using the properties data-button-title
, data-title
, data-subtitle
and data-logo
. You can customize CSS colors by using data-background-color
, data-button-color
, data-button-title-color
, data-subtitle-color
, data-terms-color
and data-title-color
.
index.html
12345678910111213141516171819202122232425262728<script type="text/javascript" src="https://resources.fidel.uk/sdk/js/v2/fidel.js" class="fidel-form" data-company-name="Your company" data-key="pk_test_demo" data-program-id="bca59bd9-171b-4d1f-92af-4b2b7305268a" data-callback="callback" data-country-code="GBR" data-metadata="metadata" data-auto-open="false" data-auto-close="false" data-allowed-countries="GBR,IRL" data-background-color="#ffffff" data-button-color="#4dadea" data-button-title="Link Card" data-button-title-color="#ffffff" data-lang="en" data-logo="https://yourcompany.com/logo.png" data-subtitle="Earn 1 point for every £1 spent online or in-store" data-subtitle-color="#000000" data-privacy-url="https://yourcompany.com/privacy" data-delete-instructions="tapping remove in your settings page." data-terms-color="#000000" data-title="Link Card" data-title-color="#000000" ></script>
You can continue using the old v1 Web SDK by setting the script
src
tohttps://resources.fidel.uk/sdk/js/v1/fidel.js
.
Fidel Web SDK Parameters
data-company-name
required- the name of the company using card-linking. Maximum length is 35 characters
data-key
required- a valid SDK Key. You can find yours in the Fidel Dashboard. It starts with `pk_`.
data-program-id
required- the id of the Fidel Program to link the card to.
data-callback
- name of the global callback function
data-country-code
values: GBR, IRL, USA, SWE- sets a country of issue for all Cards collected and removes the country select box from the UI.
data-metadata
- name of the global metadata object
data-auto-open
default: false- toggles the web form auto-open behaviour on page load
data-auto-close
default: true- toggles the web form auto-close behaviour on successful card linking
data-allowed-countries
default: all supported countries- comma separated list of countries that will be shown in the UI dropdown
data-background-color
- CSS color code of the form background
data-button-color
- CSS color code of the button background
data-button-title
- the button title. Maximum length 35 characters
data-button-title-color
- CSS color code of the button title
data-close-events
default: true- adds/removes the close button, overlay click and escape key events from the form
data-lang
default: en- the localization language to be used. The default language is en (English using British English spellings). Additional languages: en-us (English with US spelling), sv (Swedish), fr (French)
data-logo
- the logo URL of the company. Height is 35px. Extensions allowed are jpg, jpeg, png
data-subtitle
- the subtitle of the web form. Maximum length 110 characters
data-subtitle-color
- CSS color code of the subtitle
data-privacy-url
- URL of your company's privacy policy
data-delete-instructions
- user instructions to unlink card in your application
data-terms-color
- CSS color code of the terms and conditions
data-title
- the title of the web form. Maximum length 25 characters
data-title-color
- CSS color code of the title
data-scheme-*
amex / visa / mastercard:false
- disables Amex, Visa or Mastercard. Multiple can be specified, e.g.
data-scheme-mastercard: false
,data-scheme-amex: false
The data-auto-open
property allows you to open the web form automatically on page load if set to true
. If data-auto-close
is set to false
, the form won't be automatically closed after linking a card. You can set data-close-events
to false
, and the form won't add the default close events.
Fidel Web SDK Global Objects
After adding the Web SDK script on your website, a global variable Fidel
is created with two methods that you can use to open and close the web form manually, Fidel.openForm()
and Fidel.closeForm()
.
To open the iframe overlay form with a button, you could use this example:
12<button type="submit" onclick="Fidel.openForm()">Link Card</button>
Web SDK callback
To receive a callback after the form submission, you must register a Javascript global function name reference on the data-callback
property of the SDK. It will be called with the response
and error
objects after the form submits. Please see an example below:
index.html
1234567<script> function callback(error, card) { console.log("Card Link Error", error); console.log("Card Linked Successfully", card); } </script>
Web SDK metadata
To store custom data related to a Card, you can pass a Javascript global object name reference on the data-metadata
property. The metadata id
property is a non-unique index, so you can set it to a custom UID (unique identifier). Later you can query the cards using the same metadata.id
. You can use our "List Cards from Metadata ID" API Endpoint.
Hint: Adding user data in the metadata as key: value pairs can simplify reconciliation with your system. For example, adding
'myUserId':'123'
will help you match the added card to your user with id 123.
index.html
12345678<script> var metadata = { id: "this-is-the-metadata-id", myUserId: "123", customKey: "customValue", }; </script>